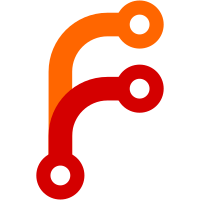
Fixes: https://codeberg.org/forgejo/forgejo/issues/820 (cherry picked from commit 6a7022ebbb83bda162974028cff01ebcc7c574ec) (cherry picked from commit 764eac47b50688d76fe90aad4819a426444ddb4a) (cherry picked from commit 1141eb7b6f2deeeca0acf1714058823d32097cfd) (cherry picked from commit 826b6509b6405ac0a0731ee0e1477ad2cbac585a) (cherry picked from commit 9990d932b8b72f9a27b6529b350eb09d44b7ef88) (cherry picked from commit 7eca57074385f296427d06c059d331d3704ccf15) (cherry picked from commit 66e1d3f082a99bb0006daf0f337850f251c235dc) (cherry picked from commit 188226a8e6b2926f1f276462741f7cc4d7a050b0) (cherry picked from commit 4cd1bff25c6cafa33464594c99b39326a6dd5740) (cherry picked from commit fad6b6d2c49492297d9d8512afc0369e544a6e75) (cherry picked from commit 5b25c3d8512466fd5fceea86b550bdb35c3aa04b) (cherry picked from commit 4746ece4dd018af781181744fb8743e83b64c6df) (cherry picked from commit 2a6f85afb33a1a0b7424c30de3cdff030f483294) (cherry picked from commit c027d724ee0b694e48d2b7ee1915ba55222a03e0) (cherry picked from commit be2f1eeaeb92e552b5defcf8b374ceb4c3a6b1ee) (cherry picked from commit 3058a54fe99c7cf0a015166b8b3f56f9ef9e45d9) (cherry picked from commit 53936d38a0cb1649748f02cf86ec684fa76825b6) (cherry picked from commit 311983cc978cc0a3128cdd8a9c12ac9605be62b9) (cherry picked from commit 1651ae757b31c31023d5e780a4446da5be8951bf) (cherry picked from commit d3dd8ea24dfd6fcf737eb16dcd0871a835b90477) (cherry picked from commit dd9d929ff0da9bdd359e20975f9cb57f835af4a4) (cherry picked from commit ed8c1a4a3674733f07ea5ff42e1a33b19b2a408c) (cherry picked from commit 4a4cb830de79406bbc1c2a3609e3c24fe5de5310) (cherry picked from commit 06a985238a033fc51ff8db2017248f5b6413af33)
350 lines
13 KiB
Go
350 lines
13 KiB
Go
// Copyright 2022 The Gitea Authors. All rights reserved.
|
|
// SPDX-License-Identifier: MIT
|
|
|
|
package auth
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
|
|
"code.gitea.io/gitea/models/perm"
|
|
)
|
|
|
|
// AccessTokenScopeCategory represents the scope category for an access token
|
|
type AccessTokenScopeCategory int
|
|
|
|
const (
|
|
AccessTokenScopeCategoryActivityPub = iota
|
|
AccessTokenScopeCategoryAdmin
|
|
AccessTokenScopeCategoryMisc // WARN: this is now just a placeholder, don't remove it which will change the following values
|
|
AccessTokenScopeCategoryNotification
|
|
AccessTokenScopeCategoryOrganization
|
|
AccessTokenScopeCategoryPackage
|
|
AccessTokenScopeCategoryIssue
|
|
AccessTokenScopeCategoryRepository
|
|
AccessTokenScopeCategoryUser
|
|
)
|
|
|
|
// AllAccessTokenScopeCategories contains all access token scope categories
|
|
var AllAccessTokenScopeCategories = []AccessTokenScopeCategory{
|
|
AccessTokenScopeCategoryActivityPub,
|
|
AccessTokenScopeCategoryAdmin,
|
|
AccessTokenScopeCategoryMisc,
|
|
AccessTokenScopeCategoryNotification,
|
|
AccessTokenScopeCategoryOrganization,
|
|
AccessTokenScopeCategoryPackage,
|
|
AccessTokenScopeCategoryIssue,
|
|
AccessTokenScopeCategoryRepository,
|
|
AccessTokenScopeCategoryUser,
|
|
}
|
|
|
|
// AccessTokenScopeLevel represents the access levels without a given scope category
|
|
type AccessTokenScopeLevel int
|
|
|
|
const (
|
|
NoAccess AccessTokenScopeLevel = iota
|
|
Read
|
|
Write
|
|
)
|
|
|
|
// AccessTokenScope represents the scope for an access token.
|
|
type AccessTokenScope string
|
|
|
|
// for all categories, write implies read
|
|
const (
|
|
AccessTokenScopeAll AccessTokenScope = "all"
|
|
AccessTokenScopePublicOnly AccessTokenScope = "public-only" // limited to public orgs/repos
|
|
|
|
AccessTokenScopeReadActivityPub AccessTokenScope = "read:activitypub"
|
|
AccessTokenScopeWriteActivityPub AccessTokenScope = "write:activitypub"
|
|
|
|
AccessTokenScopeReadAdmin AccessTokenScope = "read:admin"
|
|
AccessTokenScopeWriteAdmin AccessTokenScope = "write:admin"
|
|
|
|
AccessTokenScopeReadMisc AccessTokenScope = "read:misc"
|
|
AccessTokenScopeWriteMisc AccessTokenScope = "write:misc"
|
|
|
|
AccessTokenScopeReadNotification AccessTokenScope = "read:notification"
|
|
AccessTokenScopeWriteNotification AccessTokenScope = "write:notification"
|
|
|
|
AccessTokenScopeReadOrganization AccessTokenScope = "read:organization"
|
|
AccessTokenScopeWriteOrganization AccessTokenScope = "write:organization"
|
|
|
|
AccessTokenScopeReadPackage AccessTokenScope = "read:package"
|
|
AccessTokenScopeWritePackage AccessTokenScope = "write:package"
|
|
|
|
AccessTokenScopeReadIssue AccessTokenScope = "read:issue"
|
|
AccessTokenScopeWriteIssue AccessTokenScope = "write:issue"
|
|
|
|
AccessTokenScopeReadRepository AccessTokenScope = "read:repository"
|
|
AccessTokenScopeWriteRepository AccessTokenScope = "write:repository"
|
|
|
|
AccessTokenScopeReadUser AccessTokenScope = "read:user"
|
|
AccessTokenScopeWriteUser AccessTokenScope = "write:user"
|
|
)
|
|
|
|
// accessTokenScopeBitmap represents a bitmap of access token scopes.
|
|
type accessTokenScopeBitmap uint64
|
|
|
|
// Bitmap of each scope, including the child scopes.
|
|
const (
|
|
// AccessTokenScopeAllBits is the bitmap of all access token scopes
|
|
accessTokenScopeAllBits accessTokenScopeBitmap = accessTokenScopeWriteActivityPubBits |
|
|
accessTokenScopeWriteAdminBits | accessTokenScopeWriteMiscBits | accessTokenScopeWriteNotificationBits |
|
|
accessTokenScopeWriteOrganizationBits | accessTokenScopeWritePackageBits | accessTokenScopeWriteIssueBits |
|
|
accessTokenScopeWriteRepositoryBits | accessTokenScopeWriteUserBits
|
|
|
|
accessTokenScopePublicOnlyBits accessTokenScopeBitmap = 1 << iota
|
|
|
|
accessTokenScopeReadActivityPubBits accessTokenScopeBitmap = 1 << iota
|
|
accessTokenScopeWriteActivityPubBits accessTokenScopeBitmap = 1<<iota | accessTokenScopeReadActivityPubBits
|
|
|
|
accessTokenScopeReadAdminBits accessTokenScopeBitmap = 1 << iota
|
|
accessTokenScopeWriteAdminBits accessTokenScopeBitmap = 1<<iota | accessTokenScopeReadAdminBits
|
|
|
|
accessTokenScopeReadMiscBits accessTokenScopeBitmap = 1 << iota
|
|
accessTokenScopeWriteMiscBits accessTokenScopeBitmap = 1<<iota | accessTokenScopeReadMiscBits
|
|
|
|
accessTokenScopeReadNotificationBits accessTokenScopeBitmap = 1 << iota
|
|
accessTokenScopeWriteNotificationBits accessTokenScopeBitmap = 1<<iota | accessTokenScopeReadNotificationBits
|
|
|
|
accessTokenScopeReadOrganizationBits accessTokenScopeBitmap = 1 << iota
|
|
accessTokenScopeWriteOrganizationBits accessTokenScopeBitmap = 1<<iota | accessTokenScopeReadOrganizationBits
|
|
|
|
accessTokenScopeReadPackageBits accessTokenScopeBitmap = 1 << iota
|
|
accessTokenScopeWritePackageBits accessTokenScopeBitmap = 1<<iota | accessTokenScopeReadPackageBits
|
|
|
|
accessTokenScopeReadIssueBits accessTokenScopeBitmap = 1 << iota
|
|
accessTokenScopeWriteIssueBits accessTokenScopeBitmap = 1<<iota | accessTokenScopeReadIssueBits
|
|
|
|
accessTokenScopeReadRepositoryBits accessTokenScopeBitmap = 1 << iota
|
|
accessTokenScopeWriteRepositoryBits accessTokenScopeBitmap = 1<<iota | accessTokenScopeReadRepositoryBits
|
|
|
|
accessTokenScopeReadUserBits accessTokenScopeBitmap = 1 << iota
|
|
accessTokenScopeWriteUserBits accessTokenScopeBitmap = 1<<iota | accessTokenScopeReadUserBits
|
|
|
|
// The current implementation only supports up to 64 token scopes.
|
|
// If we need to support > 64 scopes,
|
|
// refactoring the whole implementation in this file (and only this file) is needed.
|
|
)
|
|
|
|
// allAccessTokenScopes contains all access token scopes.
|
|
// The order is important: parent scope must precede child scopes.
|
|
var allAccessTokenScopes = []AccessTokenScope{
|
|
AccessTokenScopePublicOnly,
|
|
AccessTokenScopeWriteActivityPub, AccessTokenScopeReadActivityPub,
|
|
AccessTokenScopeWriteAdmin, AccessTokenScopeReadAdmin,
|
|
AccessTokenScopeWriteMisc, AccessTokenScopeReadMisc,
|
|
AccessTokenScopeWriteNotification, AccessTokenScopeReadNotification,
|
|
AccessTokenScopeWriteOrganization, AccessTokenScopeReadOrganization,
|
|
AccessTokenScopeWritePackage, AccessTokenScopeReadPackage,
|
|
AccessTokenScopeWriteIssue, AccessTokenScopeReadIssue,
|
|
AccessTokenScopeWriteRepository, AccessTokenScopeReadRepository,
|
|
AccessTokenScopeWriteUser, AccessTokenScopeReadUser,
|
|
}
|
|
|
|
// allAccessTokenScopeBits contains all access token scopes.
|
|
var allAccessTokenScopeBits = map[AccessTokenScope]accessTokenScopeBitmap{
|
|
AccessTokenScopeAll: accessTokenScopeAllBits,
|
|
AccessTokenScopePublicOnly: accessTokenScopePublicOnlyBits,
|
|
AccessTokenScopeReadActivityPub: accessTokenScopeReadActivityPubBits,
|
|
AccessTokenScopeWriteActivityPub: accessTokenScopeWriteActivityPubBits,
|
|
AccessTokenScopeReadAdmin: accessTokenScopeReadAdminBits,
|
|
AccessTokenScopeWriteAdmin: accessTokenScopeWriteAdminBits,
|
|
AccessTokenScopeReadMisc: accessTokenScopeReadMiscBits,
|
|
AccessTokenScopeWriteMisc: accessTokenScopeWriteMiscBits,
|
|
AccessTokenScopeReadNotification: accessTokenScopeReadNotificationBits,
|
|
AccessTokenScopeWriteNotification: accessTokenScopeWriteNotificationBits,
|
|
AccessTokenScopeReadOrganization: accessTokenScopeReadOrganizationBits,
|
|
AccessTokenScopeWriteOrganization: accessTokenScopeWriteOrganizationBits,
|
|
AccessTokenScopeReadPackage: accessTokenScopeReadPackageBits,
|
|
AccessTokenScopeWritePackage: accessTokenScopeWritePackageBits,
|
|
AccessTokenScopeReadIssue: accessTokenScopeReadIssueBits,
|
|
AccessTokenScopeWriteIssue: accessTokenScopeWriteIssueBits,
|
|
AccessTokenScopeReadRepository: accessTokenScopeReadRepositoryBits,
|
|
AccessTokenScopeWriteRepository: accessTokenScopeWriteRepositoryBits,
|
|
AccessTokenScopeReadUser: accessTokenScopeReadUserBits,
|
|
AccessTokenScopeWriteUser: accessTokenScopeWriteUserBits,
|
|
}
|
|
|
|
// readAccessTokenScopes maps a scope category to the read permission scope
|
|
var accessTokenScopes = map[AccessTokenScopeLevel]map[AccessTokenScopeCategory]AccessTokenScope{
|
|
Read: {
|
|
AccessTokenScopeCategoryActivityPub: AccessTokenScopeReadActivityPub,
|
|
AccessTokenScopeCategoryAdmin: AccessTokenScopeReadAdmin,
|
|
AccessTokenScopeCategoryMisc: AccessTokenScopeReadMisc,
|
|
AccessTokenScopeCategoryNotification: AccessTokenScopeReadNotification,
|
|
AccessTokenScopeCategoryOrganization: AccessTokenScopeReadOrganization,
|
|
AccessTokenScopeCategoryPackage: AccessTokenScopeReadPackage,
|
|
AccessTokenScopeCategoryIssue: AccessTokenScopeReadIssue,
|
|
AccessTokenScopeCategoryRepository: AccessTokenScopeReadRepository,
|
|
AccessTokenScopeCategoryUser: AccessTokenScopeReadUser,
|
|
},
|
|
Write: {
|
|
AccessTokenScopeCategoryActivityPub: AccessTokenScopeWriteActivityPub,
|
|
AccessTokenScopeCategoryAdmin: AccessTokenScopeWriteAdmin,
|
|
AccessTokenScopeCategoryMisc: AccessTokenScopeWriteMisc,
|
|
AccessTokenScopeCategoryNotification: AccessTokenScopeWriteNotification,
|
|
AccessTokenScopeCategoryOrganization: AccessTokenScopeWriteOrganization,
|
|
AccessTokenScopeCategoryPackage: AccessTokenScopeWritePackage,
|
|
AccessTokenScopeCategoryIssue: AccessTokenScopeWriteIssue,
|
|
AccessTokenScopeCategoryRepository: AccessTokenScopeWriteRepository,
|
|
AccessTokenScopeCategoryUser: AccessTokenScopeWriteUser,
|
|
},
|
|
}
|
|
|
|
// GetRequiredScopes gets the specific scopes for a given level and categories
|
|
func GetRequiredScopes(level AccessTokenScopeLevel, scopeCategories ...AccessTokenScopeCategory) []AccessTokenScope {
|
|
scopes := make([]AccessTokenScope, 0, len(scopeCategories))
|
|
for _, cat := range scopeCategories {
|
|
scopes = append(scopes, accessTokenScopes[level][cat])
|
|
}
|
|
return scopes
|
|
}
|
|
|
|
// ContainsCategory checks if a list of categories contains a specific category
|
|
func ContainsCategory(categories []AccessTokenScopeCategory, category AccessTokenScopeCategory) bool {
|
|
for _, c := range categories {
|
|
if c == category {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
// GetScopeLevelFromAccessMode converts permission access mode to scope level
|
|
func GetScopeLevelFromAccessMode(mode perm.AccessMode) AccessTokenScopeLevel {
|
|
switch mode {
|
|
case perm.AccessModeNone:
|
|
return NoAccess
|
|
case perm.AccessModeRead:
|
|
return Read
|
|
case perm.AccessModeWrite:
|
|
return Write
|
|
case perm.AccessModeAdmin:
|
|
return Write
|
|
case perm.AccessModeOwner:
|
|
return Write
|
|
default:
|
|
return NoAccess
|
|
}
|
|
}
|
|
|
|
// parse the scope string into a bitmap, thus removing possible duplicates.
|
|
func (s AccessTokenScope) parse() (accessTokenScopeBitmap, error) {
|
|
var bitmap accessTokenScopeBitmap
|
|
|
|
// The following is the more performant equivalent of 'for _, v := range strings.Split(remainingScope, ",")' as this is hot code
|
|
remainingScopes := string(s)
|
|
for len(remainingScopes) > 0 {
|
|
i := strings.IndexByte(remainingScopes, ',')
|
|
var v string
|
|
if i < 0 {
|
|
v = remainingScopes
|
|
remainingScopes = ""
|
|
} else if i+1 >= len(remainingScopes) {
|
|
v = remainingScopes[:i]
|
|
remainingScopes = ""
|
|
} else {
|
|
v = remainingScopes[:i]
|
|
remainingScopes = remainingScopes[i+1:]
|
|
}
|
|
singleScope := AccessTokenScope(v)
|
|
if singleScope == "" || singleScope == "sudo" {
|
|
continue
|
|
}
|
|
if singleScope == AccessTokenScopeAll {
|
|
bitmap |= accessTokenScopeAllBits
|
|
continue
|
|
}
|
|
|
|
bits, ok := allAccessTokenScopeBits[singleScope]
|
|
if !ok {
|
|
return 0, fmt.Errorf("invalid access token scope: %s", singleScope)
|
|
}
|
|
bitmap |= bits
|
|
}
|
|
|
|
return bitmap, nil
|
|
}
|
|
|
|
// StringSlice returns the AccessTokenScope as a []string
|
|
func (s AccessTokenScope) StringSlice() []string {
|
|
return strings.Split(string(s), ",")
|
|
}
|
|
|
|
// Normalize returns a normalized scope string without any duplicates.
|
|
func (s AccessTokenScope) Normalize() (AccessTokenScope, error) {
|
|
bitmap, err := s.parse()
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
|
|
return bitmap.toScope(), nil
|
|
}
|
|
|
|
// PublicOnly checks if this token scope is limited to public resources
|
|
func (s AccessTokenScope) PublicOnly() (bool, error) {
|
|
bitmap, err := s.parse()
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
|
|
return bitmap.hasScope(AccessTokenScopePublicOnly)
|
|
}
|
|
|
|
// HasScope returns true if the string has the given scope
|
|
func (s AccessTokenScope) HasScope(scopes ...AccessTokenScope) (bool, error) {
|
|
bitmap, err := s.parse()
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
|
|
for _, s := range scopes {
|
|
if has, err := bitmap.hasScope(s); !has || err != nil {
|
|
return has, err
|
|
}
|
|
}
|
|
|
|
return true, nil
|
|
}
|
|
|
|
// hasScope returns true if the string has the given scope
|
|
func (bitmap accessTokenScopeBitmap) hasScope(scope AccessTokenScope) (bool, error) {
|
|
expectedBits, ok := allAccessTokenScopeBits[scope]
|
|
if !ok {
|
|
return false, fmt.Errorf("invalid access token scope: %s", scope)
|
|
}
|
|
|
|
return bitmap&expectedBits == expectedBits, nil
|
|
}
|
|
|
|
// toScope returns a normalized scope string without any duplicates.
|
|
func (bitmap accessTokenScopeBitmap) toScope() AccessTokenScope {
|
|
var scopes []string
|
|
|
|
// iterate over all scopes, and reconstruct the bitmap
|
|
// if the reconstructed bitmap doesn't change, then the scope is already included
|
|
var reconstruct accessTokenScopeBitmap
|
|
|
|
for _, singleScope := range allAccessTokenScopes {
|
|
// no need for error checking here, since we know the scope is valid
|
|
if ok, _ := bitmap.hasScope(singleScope); ok {
|
|
current := reconstruct | allAccessTokenScopeBits[singleScope]
|
|
if current == reconstruct {
|
|
continue
|
|
}
|
|
|
|
reconstruct = current
|
|
scopes = append(scopes, string(singleScope))
|
|
}
|
|
}
|
|
|
|
scope := AccessTokenScope(strings.Join(scopes, ","))
|
|
scope = AccessTokenScope(strings.ReplaceAll(
|
|
string(scope),
|
|
"write:activitypub,write:admin,write:misc,write:notification,write:organization,write:package,write:issue,write:repository,write:user",
|
|
"all",
|
|
))
|
|
return scope
|
|
}
|