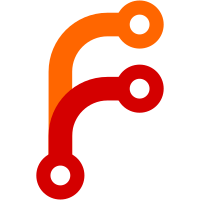
Merging PR may fail because of various problems. The pull request may have a dirty state because there is no transaction when merging a pull request. ref https://github.com/go-gitea/gitea/pull/25741#issuecomment-2074126393 This PR moves all database update operations to post-receive handler for merging a pull request and having a database transaction. That means if database operations fail, then the git merging will fail, the git client will get a fail result. There are already many tests for pull request merging, so we don't need to add a new one. --------- Co-authored-by: wxiaoguang <wxiaoguang@gmail.com> (cherry picked from commit ebf0c969403d91ed80745ff5bd7dfbdb08174fc7) Conflicts: modules/private/hook.go routers/private/hook_post_receive.go trivial conflicts because 263a716cb5 * Performance optimization for git push (#30104) was not cherry-picked and because of 998a431747a15cc95f7056a2029b736551eb037b Do not update PRs based on events that happened before they existed (cherry picked from commit eb792d9f8a4c6972f5a4cfea6e9cb643b1d6a7ce) (cherry picked from commit ec3f5f9992d7ff8250c044a4467524d53bd50210)
87 lines
2.7 KiB
Go
87 lines
2.7 KiB
Go
// Copyright 2019 The Gitea Authors. All rights reserved.
|
|
// SPDX-License-Identifier: MIT
|
|
|
|
package repository
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"strings"
|
|
|
|
repo_model "code.gitea.io/gitea/models/repo"
|
|
user_model "code.gitea.io/gitea/models/user"
|
|
"code.gitea.io/gitea/modules/setting"
|
|
)
|
|
|
|
// env keys for git hooks need
|
|
const (
|
|
EnvRepoName = "GITEA_REPO_NAME"
|
|
EnvRepoUsername = "GITEA_REPO_USER_NAME"
|
|
EnvRepoID = "GITEA_REPO_ID"
|
|
EnvRepoIsWiki = "GITEA_REPO_IS_WIKI"
|
|
EnvPusherName = "GITEA_PUSHER_NAME"
|
|
EnvPusherEmail = "GITEA_PUSHER_EMAIL"
|
|
EnvPusherID = "GITEA_PUSHER_ID"
|
|
EnvKeyID = "GITEA_KEY_ID" // public key ID
|
|
EnvDeployKeyID = "GITEA_DEPLOY_KEY_ID"
|
|
EnvPRID = "GITEA_PR_ID"
|
|
EnvPushTrigger = "GITEA_PUSH_TRIGGER"
|
|
EnvIsInternal = "GITEA_INTERNAL_PUSH"
|
|
EnvAppURL = "GITEA_ROOT_URL"
|
|
EnvActionPerm = "GITEA_ACTION_PERM"
|
|
)
|
|
|
|
type PushTrigger string
|
|
|
|
const (
|
|
PushTriggerPRMergeToBase PushTrigger = "pr-merge-to-base"
|
|
PushTriggerPRUpdateWithBase PushTrigger = "pr-update-with-base"
|
|
)
|
|
|
|
// InternalPushingEnvironment returns an os environment to switch off hooks on push
|
|
// It is recommended to avoid using this unless you are pushing within a transaction
|
|
// or if you absolutely are sure that post-receive and pre-receive will do nothing
|
|
// We provide the full pushing-environment for other hook providers
|
|
func InternalPushingEnvironment(doer *user_model.User, repo *repo_model.Repository) []string {
|
|
return append(PushingEnvironment(doer, repo),
|
|
EnvIsInternal+"=true",
|
|
)
|
|
}
|
|
|
|
// PushingEnvironment returns an os environment to allow hooks to work on push
|
|
func PushingEnvironment(doer *user_model.User, repo *repo_model.Repository) []string {
|
|
return FullPushingEnvironment(doer, doer, repo, repo.Name, 0)
|
|
}
|
|
|
|
// FullPushingEnvironment returns an os environment to allow hooks to work on push
|
|
func FullPushingEnvironment(author, committer *user_model.User, repo *repo_model.Repository, repoName string, prID int64) []string {
|
|
isWiki := "false"
|
|
if strings.HasSuffix(repoName, ".wiki") {
|
|
isWiki = "true"
|
|
}
|
|
|
|
authorSig := author.NewGitSig()
|
|
committerSig := committer.NewGitSig()
|
|
|
|
environ := append(os.Environ(),
|
|
"GIT_AUTHOR_NAME="+authorSig.Name,
|
|
"GIT_AUTHOR_EMAIL="+authorSig.Email,
|
|
"GIT_COMMITTER_NAME="+committerSig.Name,
|
|
"GIT_COMMITTER_EMAIL="+committerSig.Email,
|
|
EnvRepoName+"="+repoName,
|
|
EnvRepoUsername+"="+repo.OwnerName,
|
|
EnvRepoIsWiki+"="+isWiki,
|
|
EnvPusherName+"="+committer.Name,
|
|
EnvPusherID+"="+fmt.Sprintf("%d", committer.ID),
|
|
EnvRepoID+"="+fmt.Sprintf("%d", repo.ID),
|
|
EnvPRID+"="+fmt.Sprintf("%d", prID),
|
|
EnvAppURL+"="+setting.AppURL,
|
|
"SSH_ORIGINAL_COMMAND=gitea-internal",
|
|
)
|
|
|
|
if !committer.KeepEmailPrivate {
|
|
environ = append(environ, EnvPusherEmail+"="+committer.Email)
|
|
}
|
|
|
|
return environ
|
|
}
|