mirror of
https://0xacab.org/sutty/sutty
synced 2024-05-18 04:10:49 +00:00
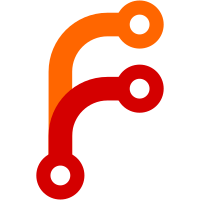
agregué el método `Jekyll::Document#reset` para que no queden datos de la versión anterior, porque jekyll mergea la información. además, para evitar bugs en las plantillas, se mantienen los arrays vacíos en el front matter para que se puedan seguir usando métodos de arrays, como each y sort.
77 lines
2.6 KiB
Ruby
77 lines
2.6 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require 'test_helper'
|
|
require_relative 'metadata_test'
|
|
|
|
class MetadataHasAndBelongsManyTest < ActiveSupport::TestCase
|
|
include MetadataTest
|
|
|
|
test 'se pueden relacionar artículos' do
|
|
author = @site.posts.create(layout: :author, title: SecureRandom.hex)
|
|
post = @site.posts.create(layout: :post, title: SecureRandom.hex)
|
|
|
|
post.authors.value = [author.uuid.value]
|
|
assert post.save
|
|
|
|
assert_includes author.posts.has_many, post
|
|
assert_includes post.authors.has_many, author
|
|
|
|
assert author.save
|
|
|
|
assert_includes author.document.data['posts'], post.document.data['uuid']
|
|
assert_includes post.document.data['authors'], author.document.data['uuid']
|
|
end
|
|
|
|
test 'se puede eliminar la relación' do
|
|
author = @site.posts.create(layout: :author, title: SecureRandom.hex)
|
|
post = @site.posts.create(layout: :post, title: SecureRandom.hex, authors: [author.uuid.value])
|
|
|
|
assert_includes post.authors.value, author.uuid.value
|
|
assert_includes author.posts.value, post.uuid.value
|
|
|
|
post.authors.value = []
|
|
assert post.save
|
|
|
|
assert_not_includes author.posts.has_many, post
|
|
assert_not_includes post.authors.has_many, author
|
|
|
|
assert_includes author.posts.had_many, post
|
|
assert_includes post.authors.had_many, author
|
|
|
|
assert author.save
|
|
|
|
assert_not_includes author.document.data['posts'], post.document.data['uuid']
|
|
assert_not_includes post.document.data['authors'], author.document.data['uuid']
|
|
end
|
|
|
|
test 'se puede cambiar la relación' do
|
|
author = @site.posts.create(layout: :author, title: SecureRandom.hex)
|
|
post1 = @site.posts.create(layout: :post, title: SecureRandom.hex, authors: [author.uuid.value])
|
|
post2 = @site.posts.create(layout: :post, title: SecureRandom.hex)
|
|
|
|
author.posts.value = [post2.uuid.value]
|
|
assert author.save
|
|
|
|
assert_not_includes author.posts.has_many, post1
|
|
assert_not_includes post1.authors.has_many, author
|
|
|
|
assert_includes author.posts.had_many, post1
|
|
assert_includes post1.authors.had_many, author
|
|
|
|
assert_not_includes author.posts.had_many, post2
|
|
assert_not_includes post2.authors.had_many, author
|
|
|
|
assert_includes author.posts.has_many, post2
|
|
assert_includes post2.authors.has_many, author
|
|
|
|
assert post1.save
|
|
assert post2.save
|
|
|
|
assert_not_includes author.document.data['posts'], post1.document.data['uuid']
|
|
assert_not_includes post1.document.data['authors'], author.document.data['uuid']
|
|
|
|
assert_includes author.document.data['posts'], post2.document.data['uuid']
|
|
assert_includes post2.document.data['authors'], author.document.data['uuid']
|
|
end
|
|
end
|